일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 | 31 |
- 안드로이드 스튜디오 인터넷 연결 안되어 있을 때
- 안드로이드 스튜디오 반복되는 레이아웃 코드
- 안드로이드 스튜디오 style
- edittext 연결
- Kotlin
- apache란
- 안드로이드 mvvm 예제
- 안드로이드 스튜디오 커스텀 다이얼로그
- 이중for문 사용 안하기
- apache nginx
- 안드로이드 디자인패턴
- 디자인 패턴 예제
- 다른 객체 리스트의 비교
- 자바 스레드 예제
- java
- java thread 예제
- 안드로이드 스튜디오 tts
- 객체지향 프로그래밍 5가지 원칙
- recyclerview item recycle
- 리사이클러뷰 아이템 재사용
- dagger error
- 변수
- 아파치 엔진엑스
- Thread
- 아파치란
- AAC
- LifeCycle
- hilt error
- 안드로이드 스튜디오 인터넷 연결 확인
- 안드로이드 스튜디오 custom dialog
- Today
- Total
Sam Story
쉐어드프리퍼런스(Sharedpreferences) 본문
SharedPreferences 란?
안드로이드 앱 개발을 하다보면 데이터를 저장해야하는 경우가 많이 생긴다.
그렇다면 이 데이터들을 어디에 어떻게 저장할까?
서버를 연동하였다면 서버의 DataBase를 사용하는 경우가 일반적이겠지만
통신을 해야된다는 하나의 절차가 더 생기게 된다.
안드로이드 내부에서 데이터를 저장하거나 불러오는 경우에는 어떤식으로 처리를 해야하는가에서
내부 DataBase인 Room 과 오늘 소개하려는 Sharedpreferences가 대표적으로 이용된다.
그중에서도 내부 DataBase인 Room의 경우는 아무래도 기본적인 쿼리문에 대한 이해와 데이터베이스의
구조에 대해 이해를 하고 있어야 사용하는데 크게 제약이 될것이 없다.
이러한 내부,서버쪽 DataBase보다 간단하게 데이터를 관리할 수 있는게 SharedPreferences 이다.
오늘은 이 SharedPreferences를 이용하여 간단한 회원가입 로그인 기능을 만들어 보도록 하겠다.
(※ ※ 로그인 상황이나 회원가입 상황에서 여러 예외처리는 생략되어 있음 ※ ※ )
ex) 공백일 때도 Shared에 저장이 되니 참고
예제
관련 내용들에 필요한 주석을 달아놨음.
메인 액티비티 레이아웃( 로그인 화면 레이아웃 )
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<TextView
android:padding="50dp"
android:layout_gravity="center"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="로그인 화면"
android:textSize="40sp"
/>
<EditText
android:id="@+id/editTextInputLoginId"
android:layout_marginHorizontal="20dp"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="아이디"/>
<EditText
android:id="@+id/editTextInputLoginPassword"
android:layout_marginTop="20dp"
android:layout_marginHorizontal="20dp"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="비밀번호"/>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:gravity="center">
<Button
android:id="@+id/buttonLogin"
android:layout_margin="10dp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="로그인"
/>
<Button
android:id="@+id/buttonSignUp"
android:layout_margin="10dp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="회원가입"
/>
</LinearLayout>
</LinearLayout>
메인 액티비티
package com.example.sharedexample
import android.content.Intent
import android.content.SharedPreferences
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.widget.Button
import android.widget.EditText
import android.widget.Toast
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// MainActivity View 초기화
val editTextInputLoginId: EditText = findViewById(R.id.editTextInputLoginId)
val editTextInputLoginPassword: EditText = findViewById(R.id.editTextInputLoginPassword)
val buttonLogin: Button = findViewById(R.id.buttonLogin)
val buttonSignUp: Button = findViewById(R.id.buttonSignUp)
// SharePreferences를 초기화 해주고 저장한 KEY 값으로 불러온다.
val preferences: SharedPreferences = getSharedPreferences("userInfo", MODE_PRIVATE)
// 로그인 버튼 클릭리스너
buttonLogin.setOnClickListener {
//첫번째로 아이디가 일치하는지 확인한다.
when(editTextInputLoginId.text.toString()){
preferences.getString("id","") -> {
// 두번째로 비밀번호가 일치하는지 확인
when(editTextInputLoginPassword.text.toString()){
preferences.getString("password","") ->
// 확인 후 아이디,비밀번호 둘다 일치하면 토스트 메시지를 띄워준다.
Toast.makeText(this,"로그인에 성공하셨습니다.",Toast.LENGTH_SHORT).show()
// 비밀번호가 일치하지 않는 경우 토스트 메시지를 띄워준다.
else -> Toast.makeText(this,"비밀번호가 일치하지 않습니다.",Toast.LENGTH_SHORT).show()
}
}
// 아이디가 일치하지 않는경우
else -> Toast.makeText(this,"아이디가 일치하지 않습니다.",Toast.LENGTH_SHORT).show()
}
}
// 회원가입 버튼 클릭 리스너로 SubActivity로 진입한다.
buttonSignUp.setOnClickListener {
val intent = Intent(this,SubActivity::class.java)
startActivity(intent)
}
}
}
서브 액티비티 레이아웃 ( 회원가입 화면 레이아웃 )
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".SubActivity">
<TextView
android:padding="50dp"
android:layout_gravity="center"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="회원가입 화면"
android:textSize="40sp"
/>
<EditText
android:id="@+id/editTextInputSignUpId"
android:layout_marginHorizontal="20dp"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="아이디"/>
<EditText
android:id="@+id/editTextInputSignUpPassword"
android:layout_marginTop="20dp"
android:layout_marginHorizontal="20dp"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="비밀번호"/>
<Button
android:id="@+id/buttonSignUpCheck"
android:layout_gravity="center"
android:layout_margin="10dp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="확인"
/>
</LinearLayout>
서브 액티비티
package com.example.sharedexample
import android.content.SharedPreferences
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.widget.Button
import android.widget.EditText
import android.widget.Toast
class SubActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_sub)
// 액티비티에서 쓰이는 View 초기화
val editTextInputSignUpId: EditText = findViewById(R.id.editTextInputSignUpId)
val editTextInputSignUpPassword: EditText = findViewById(R.id.editTextInputSignUpPassword)
val buttonSignUpCheck: Button = findViewById(R.id.buttonSignUpCheck)
// getSharedPreferences("파일이름",'모드')
// 모드 == 0 (읽기,쓰기가능)
// 모드 == MODE_PRIVATE (이 앱에서만 사용가능)
val preferences: SharedPreferences = getSharedPreferences("userInfo", MODE_PRIVATE);
// 확인버튼 눌렀을때
buttonSignUpCheck.setOnClickListener {
// editor를 초기화 해준다.
val editor: SharedPreferences.Editor = preferences.edit()
// editor를 이용해 KEY , VALUE 값으로 put해주는 메서드
editor.putString("id",editTextInputSignUpId.text.toString())
editor.putString("password",editTextInputSignUpPassword.text.toString())
// editor를 이용해 처리를했으면 apply() or commit() 을 해주어야한다.
editor.apply()
// 회원가입 완료 후 토스트메시지 띄워준 후 액티비티 종료
Toast.makeText(this,"회원가입이 완료되었습니다",Toast.LENGTH_SHORT).show()
finish()
}
}
}
실행 화면
회원가입에서 간단한 내용을 입력 후 회원가입을 한 후
로그인 화면에서 로그인을 하게 되면
위에 이미지 처럼 로그인에 성고했다는 toast메시지를 볼 수 있다.
그리고 이런 쉐어드에 저장되어 있는 데이터를 코드로 보고싶다면
안드로이드 우측에 Device File Manager 탭에서 아래의 경로로 들어간 후
파일 경로 = data/data/(package_name)/shared_prefs/SharedPreference
폴더를 보면 userInfo로 저장한 xml 파일이 있을 것이다
그파일을 열어보게 되면
<?xml version='1.0' encoding='utf-8' standalone='yes' ?>
<map>
<string name="id">sam</string>
<string name="password">123</string>
</map>
현재 SharedPreferences에 저장되어 있는 KEY , VALUE 값을 확인해 볼 수 있다.
이러한 SharedPreferences를 활용하면 간단한 데이터들에 대한 처리를 쉽고 간편하게 할 수 있다.
이를 이용한 기능들도 다양하게 만들어 볼 수 있었다.
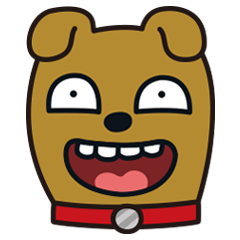
'Android' 카테고리의 다른 글
레트로핏 (Retrofit) (0) | 2024.03.26 |
---|---|
뷰 바인딩 (ViewBinding) (0) | 2024.03.19 |
Visibility 속성 (2) | 2024.03.16 |
리사이클러뷰(RecyclerView) (1) | 2024.03.12 |
Activity 생명주기(Activity LifeCycle) (0) | 2024.03.08 |